File organization can be tough, especially as the number of stored files gets bigger. Thankfully, the Dropbox API has a robust, configurable search endpoint that allows you to quickly get to the files you need.
Last summer, Dropbox released an updated search endpoint. The new /files/search_v2 endpoint has a wide range of useful features that make it more useful than older v1 version. In this blog post, we’ll cover some of the cool stuff you can do with these new search features.
We’ll be retiring the old v1 search on October 28th, 2021. If you haven’t already, please migrate to the search v2 endpoint at your earliest convenience.
New Search Features
Improved performance and reliability
The new version of search has over 20% performance improvement compared to v1. Additionally, the reliability of search v2 is improved compared to v1 and /files/search_v2 is being consumed internally for our own surfaces. Moving from v1 to v2 search will have a positive impact on your app performance!
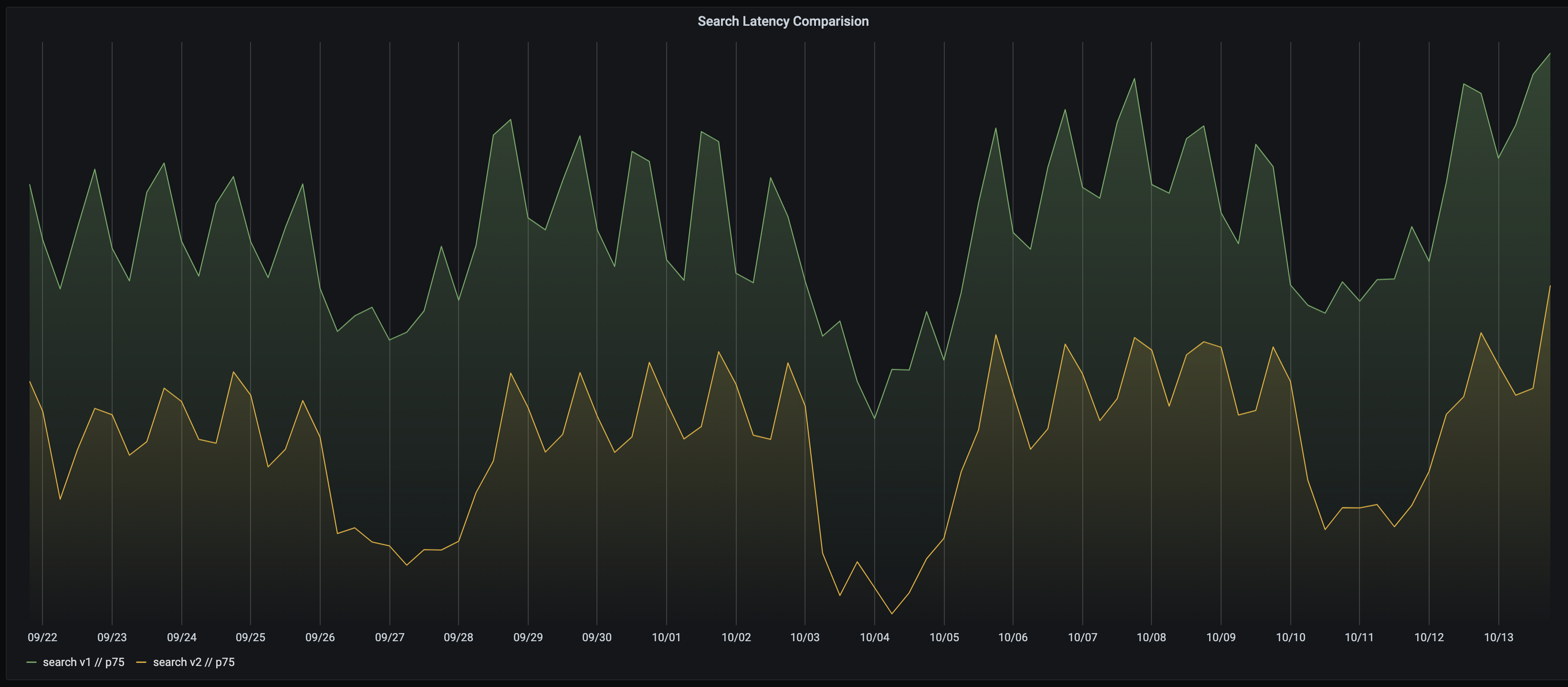
Search across more file types
The /files/search_v2 endpoint returns more file types that are supported by Dropbox. That includes Paper files, “online-only” files like G Suite or O365, and unmounted files. Unmounted files are files that have been shared with the API caller but are not “mounted” to their Dropbox. You can read more about mounting and unmounting behavior in our Sharing Guide.
Filter results by file extension
Only care about a certain type of file? Use search options to restrict your search to specific file extensions. If you use the file_extensions parameter to define a list of extensions (i.e. ["pdf", "xlsx", "mp3"]), then only files that match those extensions will be returned.
Restrict search to specific file categories
Using the search options, you can specify a list of file_categories such as ["spreadsheet", "presentation"]. Now your search results will only include file types in the specified categories.
Order results results by last modified time
By default, search results are sorted based on relevance. However, search v2 offers new functionality that allows you to sort results based on the last time a file was modified. You can turn this on by setting order_by to last_modified_time in your search options.
Improved pagination scheme
The /files/search/continue_v2 endpoint uses a cursor returned by /files/search_v2 so you can paginate between results.
You can test the pagination behavior by limiting your number of results with max_results:
curl -X POST 'https://api.dropboxapi.com/2/files/search_v2' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <YOUR_ACCESS_TOKEN>' \
--data '{
"query": "cats",
"options": {
"max_results":1
}
}'
Now you can use the returned cursor to get the next page of search results.
curl -X POST https://api.dropboxapi.com/2/files/search/continue_v2 \
--header "Authorization: Bearer <YOUR_ACCESS_TOKEN>" \
--header "Content-Type: application/json" \
--data "{\"cursor\": \"ZtkX9_EHj3x7PMkVuFIhwKYXEpwpLwyxp9vMKomUhllil9q7eWiAu\"}"
Include filename highlights in the search results
When using the Dropbox user interface to search for a file, the results highlight exact matches for the search string.
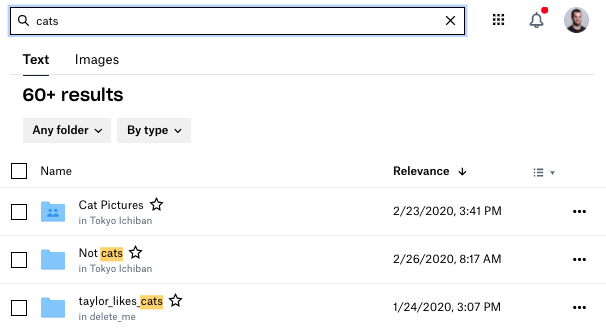
When calling /files/search_v2, use the include_highlights Boolean to optionally return the highlighted spans with search results:
{
"has_more": false,
"matches": [
{
"highlight_spans": [
{
"highlight_str": "Not ",
"is_highlighted": false
},
{
"highlight_str": "cats",
"is_highlighted": true
}
],
"metadata": {
".tag": "metadata",
"metadata": {
".tag": "folder",
"id": "id:7AObMBUU53AAAAAAAAAAbw",
"name": "Bad cats",
"path_display": "/Tokyo Ichiban/Not cats",
"path_lower": "/tokyo ichiban/not cats",
"property_groups": []
}
}
},
...
]
}
Samples: Using the New Search
Search by file category:
curl -X POST https://api.dropboxapi.com/2/files/search_v2 \
--header "Authorization: Bearer <YOUR_ACCESS_TOKEN>" \
--header "Content-Type: application/json" \
--data '{"query":"forecast", "options":{"file_categories":["spreadsheet"]}}'
{
"has_more":false,
"matches":[
{
"metadata":{
".tag":"metadata",
"metadata":{
".tag":"file",
"name":"test.xlsx",
"path_display":"/Finance/Financial_Forecast_2020.xlsx",
"path_lower":"/finance/financial_forecast_2020.xlsx",
"client_modified":"2020-09-29T00:32:28Z",
"content_hash":"e7b8bc84143e3b3b2e11e0f8249a8e7d8164d729a355f16cda3d2ce097f3f6c9",
"has_explicit_shared_members":false,
"id":"id:AxJ4LfEkRbAAAAAAAAADHw",
"is_downloadable":true,
"rev":"015b068eb0fd51b000000011d5cd060",
"server_modified":"2020-09-29T00:32:30Z",
"sharing_info":{
"modified_by":"dbid:AAD6Wt3CKJD3BQJ03sdip12NDyvpqIPxlfU",
"parent_shared_folder_id":"4787589216",
"read_only":false
},
"size":8162
}
}
},
...
]
}
Search by file extension
Imagine you’re trying to find photos of whiteboarding sessions in your Dropbox files. Your team has a new whiteboarding session each month, but there isn’t a formal process around who takes the photo or where it goes.
A good way to find them would be by limiting your search to specific file extensions and ordering by last modification time.
Request sample:
curl -X POST https://api.dropboxapi.com/2/files/search_v2 \
--header "Authorization: Bearer <YOUR_ACCESS_TOKEN>" \
--header "Content-Type: application/json" \
--data '{
"query":"whiteboard",
"options": {
"file_extensions": ["jpg", "jpeg", "png"],
"order_by": "last_modified_time"
},
"match_field_options": {
"include_highlights": true
}
}'
Response sample:
{
"has_more": false,
"matches": [
{
"highlight_spans": [
{
"highlight_str": "Nov_2020_",
"is_highlighted": false
},
{
"highlight_str": "whiteboard",
"is_highlighted": true
},
{
"highlight_str": "ing",
"is_highlighted": false
}
],
"metadata": {
".tag": "metadata",
"metadata": {
".tag": "file",
"client_modified": "2020-10-12T23:41:35Z",
"content_hash": "404b4b648afe28177cbb9bf18e1fdb694d306095c81edb8d9a7be360df2303c6",
"has_explicit_shared_members": false,
"id": "id:7AObMBEU53AAAAAAAAAbw",
"is_downloadable": true,
"name": "Nov_2020_whiteboarding.jpg",
"path_display": "/Brainstorming/Nov_2020_whiteboarding.jpg",
"path_lower": "/brainstorming/nov_2020_whiteboarding.jpg",
"property_groups": [],
"rev": "015b181d6c47362000000017daa20d0",
"server_modified": "2020-10-12T23:41:35Z",
"sharing_info": {
"modified_by": "dbid:AABuXdtqA88UpveXxu7rcTSo64ADcrWnBMk",
"parent_shared_folder_id": "6403268816",
"read_only": false
},
"size": 65353
}
}
},
{
"highlight_spans": [
{
"highlight_str": "Whiteboard",
"is_highlighted": true
},
{
"highlight_str": "ing ",
"is_highlighted": false
},
{
"highlight_str": "Oct2020",
"is_highlighted": false
}
],
"metadata": {
".tag": "metadata",
"metadata": {
".tag": "file",
"client_modified": "2020-03-04T20:54:47Z",
"content_hash": "bc8f3f67578f1a9926c0bd0f93f4068ea74745af079e4837ca3ae53d253f1b9f",
"has_explicit_shared_members": false,
"id": "id:7AObMBEU53AAAAAAAAAbw",
"is_downloadable": true,
"name": "Whiteboarding Oct2020.png",
"path_display": "/Q4_ideas/Whiteboarding Oct2020.png",
"path_lower": "/q4_ideas/whiteboarding oct2020.png",
"property_groups": [],
"rev": "015a00da174f18d000000017daa20d0",
"server_modified": "2020-03-04T20:54:47Z",
"sharing_info": {
"modified_by": "dbid:AABuXdtqA88UpveXxu7rcTSo64ADcrWnBMk",
"parent_shared_folder_id": "6403268816",
"read_only": false
},
"size": 116317
}
}
}
...
]
}
Migrating to the new search
The /files/search_v2 endpoint offers some powerful new features to help you more easily grab what you need from Dropbox. In addition to better performance, the new search endpoint includes more supported file types in the results, allows you to filter by extension or category, and adds other features like pagination and highlighting. We’ll be retiring search v1 on Feb 28th, 2021 so we recommend migrating if your app depends on search functionality. As always, we’re available to help! You can post your questions on our developer forum or submit a ticket for more direct support.
Build with Dropbox today at www.dropbox.com/developers.