Dropbox (and the Dropbox API) has recently expanded the flexibility of our permissions model by adding the ability for Dropbox Business users to create folders that have a more restricted audience than their parent folders. This is perfect for cases where you want a sub-folder to have a smaller audience* than its parent folder.
*Note: this article is focused on restricted access use cases, but it is possible to create sub-folders that have more shares than a parent folder.
In this article, we’ll cover details of what it means to restrict folder permissions, how to edit those permissions using the Dropbox API, and run through some common use cases.
What does it mean for a folder to have restricted access?
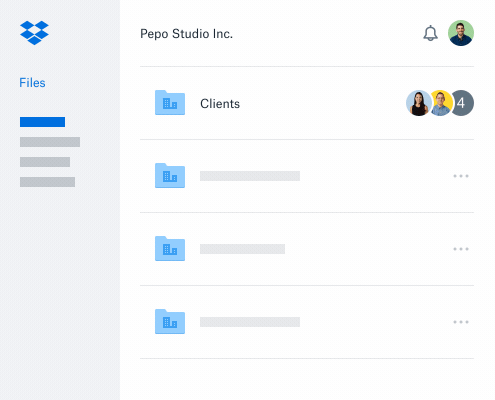
By default, new shared folders inside a team folder inherit their share settings. However, folders with restricted access do not inherit member access from their parent folder, and can instead be set to a more limited audience. Once the inheritance has been broken, you can add specific individual members or groups to customize who has access (and at what level) to the specific sub-folder. These types of restricted folders can only be created inside team folders.
Note: the “Dropbox Web” instructions in this article are meant to help follow along, but are subject to change. For official instructions please refer to the restrict folder access help center article.
How do I restrict access to a folder?
New Folder
Dropbox Web: Inside a team folder, click on “New folder” from the web page, and select the “Specific people” option from the subsequent modal pop up.
Dropbox API: Using the /sharing/share_folder endpoint you can designate a path and name for the new folder (one will be created if the path
is not found). Setting access_inheritance
to no_inherit
will ensure the resulting folder is created without any inherited permissions. You can then customize who has access to the folder with /sharing/add_folder_member, which accepts a dropbox_id for an account, team member, or a group.
Existing Folders
Dropbox Web: From the sharing modal you can restrict access by removing the inherited members of the parent folder. You can either remove all* the inherited members of the parent folder at once, or remove one inherited user/group at a time.
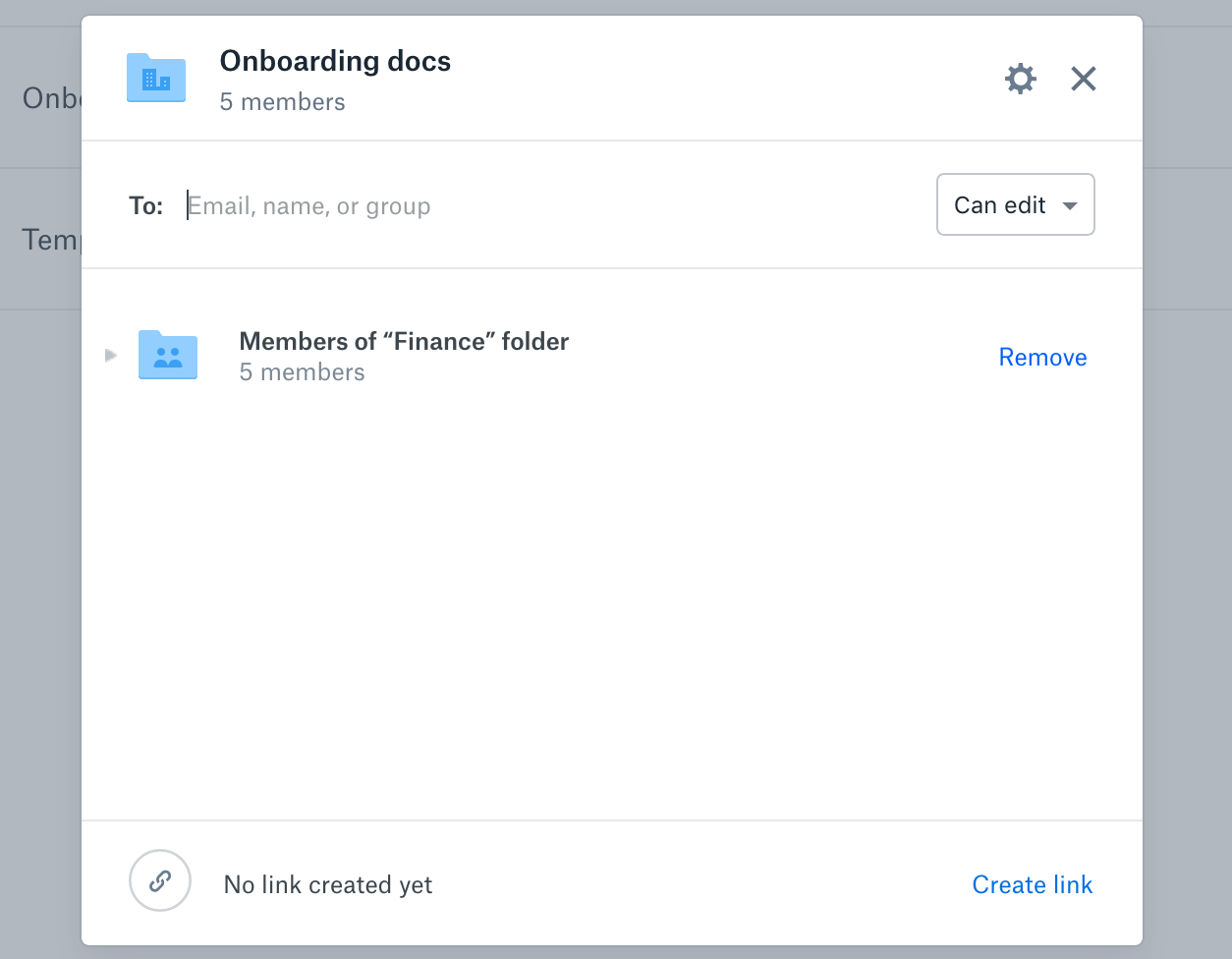
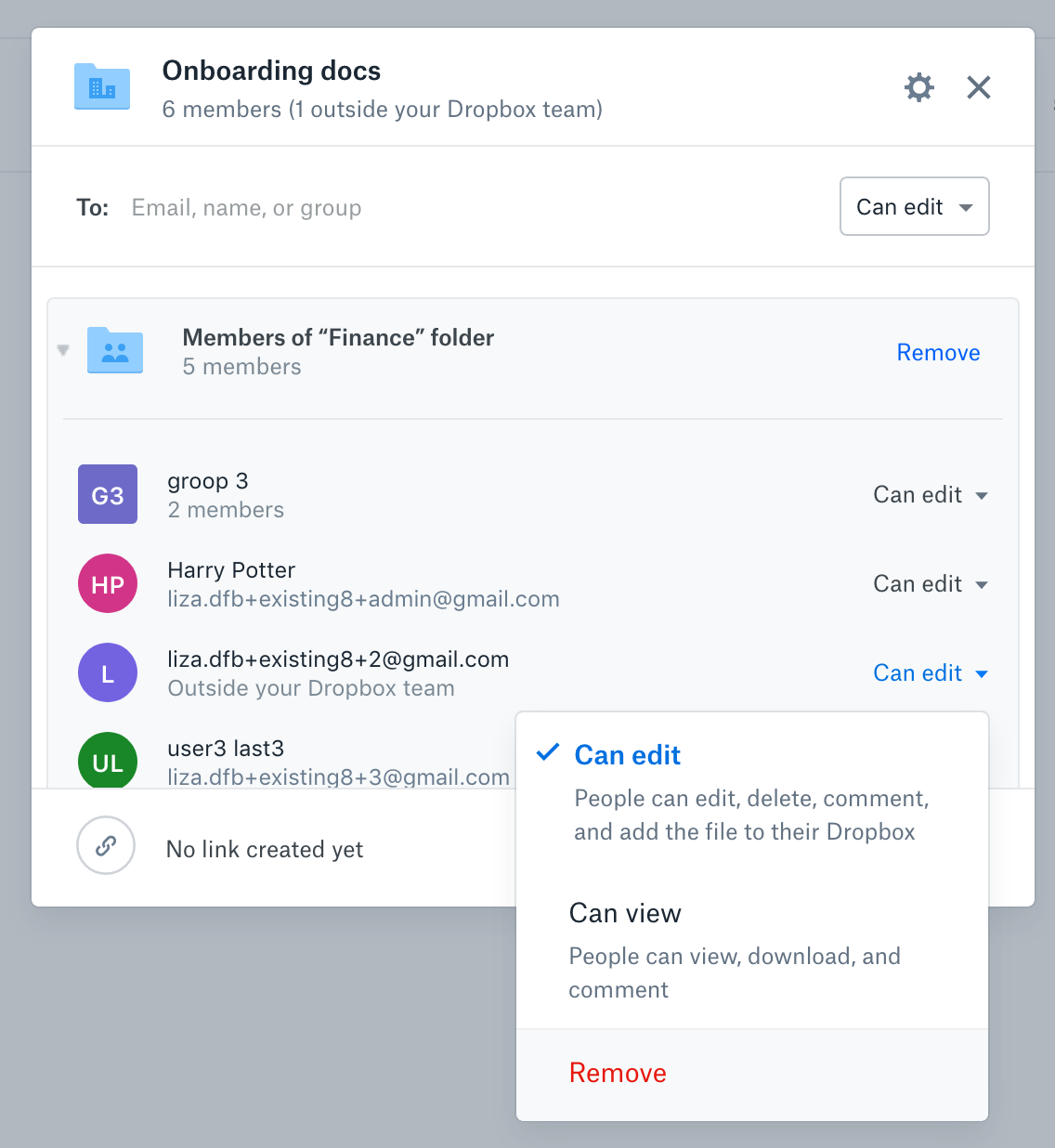
Dropbox API: Use the /sharing/set_access_inheritance endpoint to pass in the shared_folder_id
and no_inherit
for the access_inheritance
parameter. Once executed, this breaks the inherited permissions from the parent folder and removes all* current groups and individuals with access to the folder. From here you can assign a custom permission set by calling sharing/add_folder_member and adding specific groups or people.
*Currently you cannot remove inherited members from an existing folder with 100+ members.
How do I restore parent folder members?
Dropbox Web: Inherited members can be restored by clicking on “share with members” in the gray banner. Once parent folder members are restored, the folder no longer has restricted access.
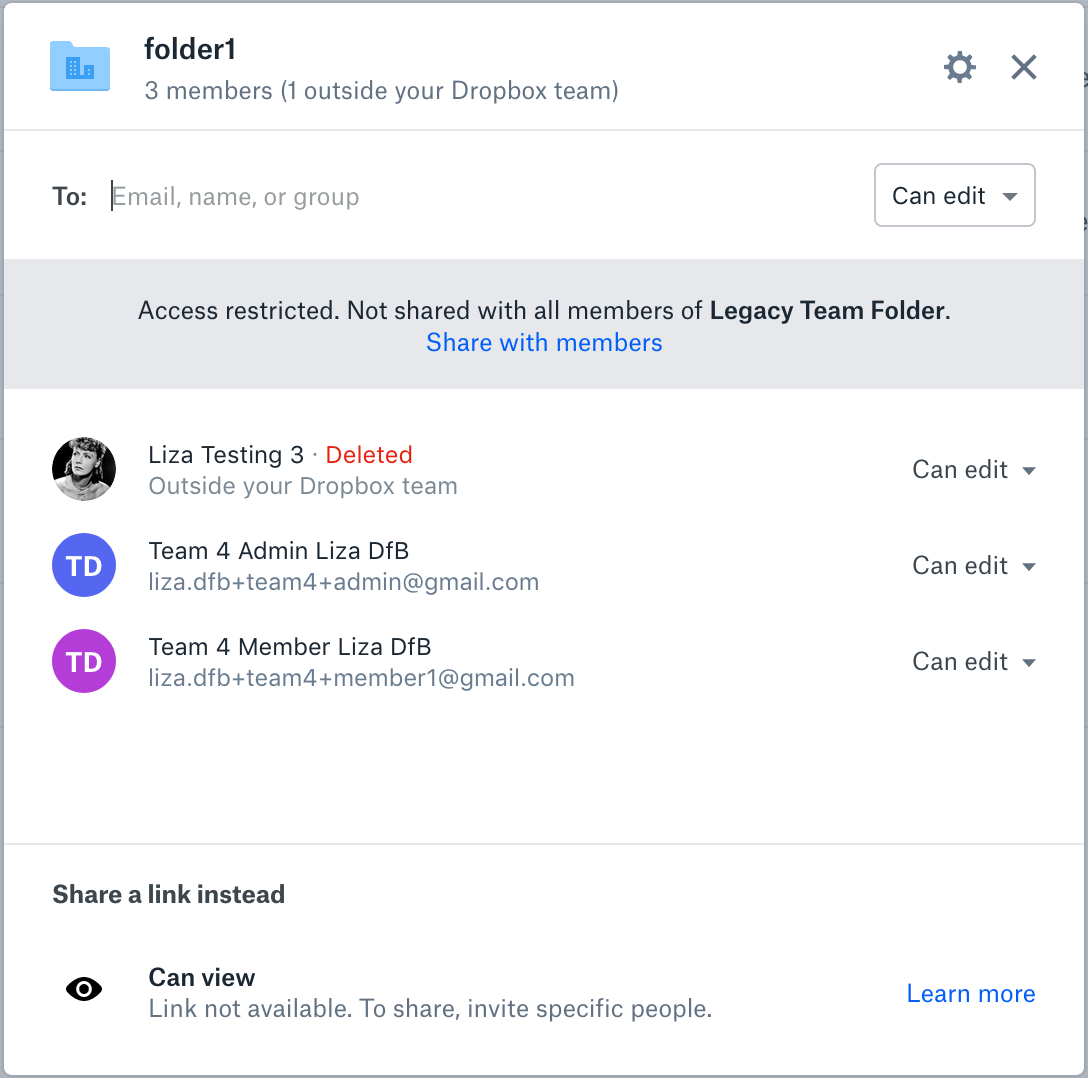
Dropbox API: Executing the sharing/set_access_inheritance endpoint with the shared_folder_id and and passing the setting inherit will restore all inherited parent folder members.
Use Cases
Now that we have a good overview of how nested permissions and restricted folders work, let’s dive into an implementation scenario with the Dropbox API.
Imagine you’re an admin for a media company. You’re setting up a secret new project folder for one of your existing clients. Because the client’s product launch is highly-confidential, only certain members of the existing client team will be working on this new project. You want to make sure all client assets are organized under the right hierarchy but want to limit who has access to the specific project folder to keep the launch under wraps. Fortunately, restricted folder permissions allow you to do exactly that.
Creating your folder
Create the project folder underneath the client folder and restrict permissions using the /sharing/share_folder endpoint.
curl -X POST https://api.dropboxapi.com/2/sharing/share_folder \
--header "Authorization: Bearer <team_file_access_token> " \
--header "Content-Type: application/json" \
--header "Dropbox-API-Select-Admin: dbmid:AACf3TCu4HhXLhlYmfbrWnV3AvkQ-0oxCLp" \
--data "{\"path\": \"/Client X/Secret Project Folder\",
\"acl_update_policy\": \"editors\",
\"force_async\": false,
\"member_policy\": \"team\",
\"shared_link_policy\": \"members\",
\"access_inheritance\": \"no_inherit\"}"
While you also have more granular options for setting folder policies, setting access_inheritance to no_inherit in this context will create a folder with restricted access that does not mirror parent permissions. Providing a path that does not already exist will create the folder specified, otherwise it will create a new share for the existing path and return a shared_folder_id.
Setting permissions
Next we want to grant access to the secret project folder for a specific client team (already set up as a Dropbox Group). First, let’s grab the shared_folder_id from the return of the above call or using /files/get_metadata. From here you can pass in the shared_folder_id and specify a dropbox_id to grant access to a specific group of team members with the /sharing/add_folder_member endpoint. In this example, the secret client team has already been added to a group, and we’re adding that group to the folder with the group’s dropbox_id. Although we’re adding a group in this call, a dropbox_id could belong to a Dropbox account, individual team member, or a group.
curl -X POST https://api.dropboxapi.com/2/sharing/add_folder_member \
--header "Authorization: Bearer <team_file_access_token>" \
--header "Content-Type: application/json" \
--header "Dropbox-API-Select-Admin: dbmid:AACf3TCu4HhXLhlYmfbrWnV3AvkQ-0oxCLp" \
--data "{\"shared_folder_id\": \"6448417792\",
\"members\": [{\"member\":
{\".tag\": \"dropbox_id\",
\"dropbox_id\": \"g:76264835542b7610000000000000219e\"},
\"access_level\": \"editor\"}],
\"quiet\": true}"
Adjusting permissions
Oh no! You accidentally added the wrong members to your restricted folder. No worries, first let’s look at all the current folder members with /sharing/list_folder_members. Then we can decide what groups or members to remove.
curl -X POST https://api.dropboxapi.com/2/sharing/list_folder_members \
--header "Authorization: Bearer <team_file_access_token>" \
--header "Content-Type: application/json" \
--header "Dropbox-API-Select-Admin: dbmid:AACf3TCu4HhXLhlYmfbrWnV3AvkQ-0oxCLp" \
--data "{\"shared_folder_id\": \"6448417792\",
\"actions\": []}"
Once you’ve identified the group or member you want to remove, from the response of the /sharing/list_folder_members call, you can go ahead and use /sharing/remove_folder_member. Make sure to pass in the relevant dropbox_id of members (or groups) that you want to remove and the shared_folder_id for the target folder.
curl -X POST https://api.dropboxapi.com/2/sharing/remove_folder_member \
--header "Authorization: Bearer <team_file_access_token>" \
--header "Content-Type: application/json" \
--header "Dropbox-API-Select-Admin: dbmid:AACf3TCu4HhXLhlYmfbrWnV3AvkQ-0oxCLp" \
--data "{\"shared_folder_id\": \"6448417792\",
\"member\": {\".tag\": \"dropbox_id\",
\"dropbox_id\": \"g:76264835542b7610000000000000219e\"},
\"leave_a_copy\": false}"
Restoring permissions
Great, now the project team has had time to successfully execute their project in secret. They’ve delivered the project, the client loved it, the product was launched, and now you want to restore the default permissions so the rest of the client team can leverage the great work they did! You can do this by calling /sharing/set_access_inheritance and passing in inherit. This will revert permissions back to the defaults of the parent folder.
curl -X POST https://api.dropboxapi.com/2/sharing/set_access_inheritance \
--header "Authorization: Bearer <team_file_access_token>" \
--header "Content-Type: application/json" \
--header "Dropbox-API-Select-User: dbmid:AACf3TCu4HhXLhlYmfbrWnV3AvkQ-0oxCLp" \
--data "{\"shared_folder_id\": \"6448417792\",
\"access_inheritance\": \"inherit\"}"
Common Questions
Who can restrict access to a folder?
Any member of the team can restrict access to a folder, as long as they have the ability to manage membership of that folder.
What error messages do users see when accessing a restricted folder?
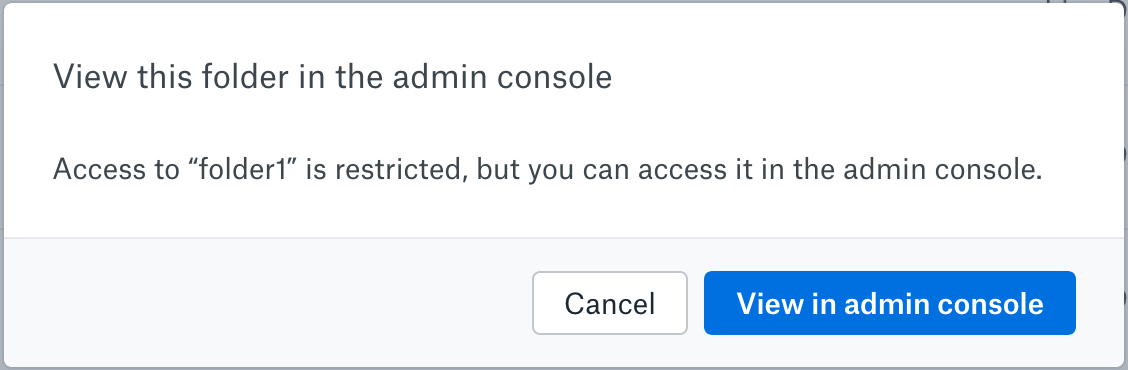
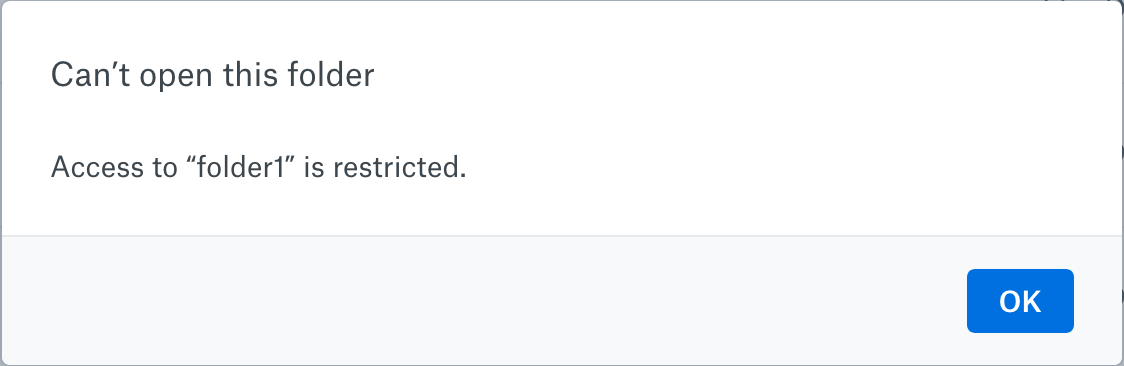
How is this feature visible in Dropbox?
Icon | Meaning |
![]() |
A user who does not have access to a folder in a team folder will see this icon on all surfaces. |
![]() |
A user who does not have access to a folder in a team folder, but is added to one or more subfolders, will see this icon on all surfaces. They can click into this folder to get to the subfolders they have access to, but they will not see other content in this folder. |
![]() |
A user who has access to a folder within a team folder will see this icon on all surfaces. |
Best practices for large deployments
⚠️ Avoid creating multiple folders with restricted access at the same time. Nothing will break, but you may encounter a namespace lock contention. - E.g. Avoid using our APIs to mass-create folders with restricted access in the same folder tree.
⚠️ Continue to adhere to our guidance that a team should not have more than 10,000 shared folders. A folder you restrict access to is always a shared folder. Dropbox performance may decline for teams with over 10,000 shared folders.
⚠️ Try to keep team folders flat when possible, rather than having deeply nested sharing structures. Dropbox performance may decline for large teams with deeply nested structures.
⚠️ Don’t restrict access to folders with 100+ members. Nothing will break, but you will see a generic error message. An interim workaround is to create a new restricted folder and move the contents of an existing folder into the newly created folder.
Sharing with the Dropbox API
In this article, we covered what it means to restrict folder permissions, how to edit those permissions (in the Dropbox user interface and the Dropbox API), discussed a real world use case, and gave some context for working with larger scale deployments. Between this post, ‘3 ways to add Sharing to your Dropbox App’, and ‘Manage team sharing in your Dropbox App’, we’ve covered many Dropbox Sharing concepts and how to incorporate them into your own integration. Armed with this new knowledge you can move beyond simple file storage to collaboration and administration. We can’t wait to see what you build!
See what’s possible with the Dropbox API and find other ways to automate your work.
Build with Dropbox today at www.dropbox.com/developers